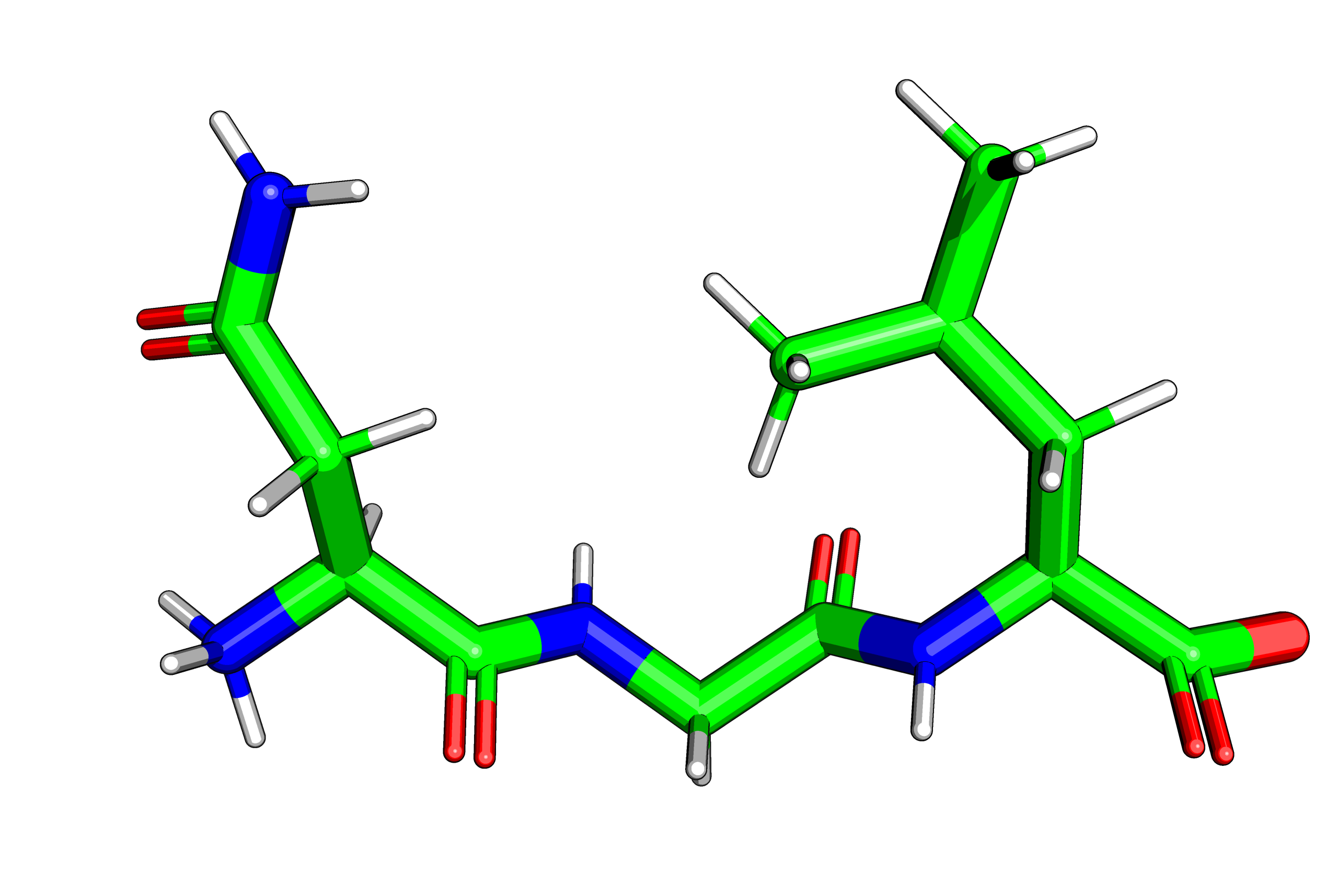
Michelanglo — Documentation
API
Python API
A clientside python 3 API is provided at MichelaNGLo-api .
The code below describes the basics of how to interact with the site without it.
Step one: get cookie
A post request to /login
with the parameters {'username': 'xxxx', 'password': 'xxxx', 'action': 'login'}
, will reply with a response with Set-Cookie
, which you will add to your request headers as Cookie
as normal.
If you use python requests.Session()
, this is handled automatically.
##python3 from warnings import warn import requests mike = requests.Session() base_url = 'https://michelanglo.sgc.ox.ac.uk' data = {'username': 'yournamehere', 'password': 'yourpasswordhere', 'action': 'login'} r = mike.post(base_url+'/login', data=data)
If you are somehow struggling with cookies or what to check if you are logged in the payload {'action': 'whoami'}
to /login
, will reply with your username.
Get uuid of your pages
get_pages
returns a dictionary of arrays of uuid of your pages, with keys 'owned', 'visited' and 'public'. Note that if not logged in, you get the following error: {"owned": "not logged in", "visited": "not logged in", "error": "not logged in", "public": []}
Get protein page as json
Adding mode: json
to the request for a protein page will return a json, either as a post or get request (you can try the latter in your browser by adding ?mode=json
).
If you are using an encrypted page you can only get the data back by providing key: password
.
The data is encrypted with the hash of the password and this is all handled serverside, so the password is the human readable password, not the token.
Edit page
data = {'page': 'the-long-uuid-for-the-page', 'confidential': 'true', 'public': 'false', 'encryption': 'false', 'columns_viewport': 6, 'columns_text': 6, 'title': 'New title', 'description': 'New markdown text', 'loadfun': loadfun } r = mike.post(base_url+'/edit_user-page', data=data) print(r.content)
To prevent XSS threats (example), loadfun
and script
tags in the description (or title) are forbidden for regular users —email the site admin to discuss alternatives.
Example
Let's copy the content of one page into another (note this can be done in the main page)
#python3 import requests, json donor_url = '00000000-0000-1000-a000-000000000000' recipient_url = '00000000-0000-1000-a000-000000000001' mike = requests.Session() base_url = 'https://michelanglo.sgc.ox.ac.uk' data = {'username': 'yournamehere', 'password': 'yourpasswordhere', 'action': 'login'} r = mike.post(base_url+'/login', data=data) donor = json.loads(mike.post(base_url+'/data/'+donor_url, data={'mode':'json'}).content.decode('utf-8')) donor['page'] = mash_url print(mike.post(base_url+'/edit_user-page', data=donor).content) donor = json.loads(mike.post(base_url+'/data/'+donor_url, data={'mode':'json'}).content.decode('utf-8')) mash = json.loads(mike.post(base_url+'/data/'+mash_url, data={'mode':'json'}).content.decode('utf-8')) for key in donor: if donor[key] != mash[key]: if isinstance(donor[key],bool): print(f'Difference with {key} {type(donor[key]).__name__}:{donor[key]} vs. {type(mash[key]).__name__}{mash[key]}') else: print(f'Difference with {key} {type(donor[key]).__name__}:{len(donor[key])} vs. {type(mash[key]).__name__}:{len(mash[key])}')